Python Data Types
- Dev Deck
- Nov 9, 2020
- 9 min read
In general, a data type defines the format, sets the upper & lower bounds of the data so that a program could use it appropriately. However, Python data types are just more than that. In Python, we don’t need to declare a variable with explicitly mentioning the data type. This feature is famously known as dynamic typing.
Python determines the type of a literal directly from the syntax at runtime. For example – the quotes mark the declaration of a string value, square brackets represent a list and curly brackets for a dictionary. Also, the non-decimal numbers will get assigned to Integer type whereas the ones with a decimal point will be a float.
Everything including variables, functions, modules in Python is an object. Another interesting fact is that variables don’t have types instead they are just labels in Python. It is the value which gets associated with a type. Hence, the same variable, the label can refer values of different Python data types.
Below is the list of important data types that are commonly used in Python. We’ll discuss each of them with examples.
Booleans
Numbers
Strings
Bytes
Lists
Tuples
Sets
Dictionaries
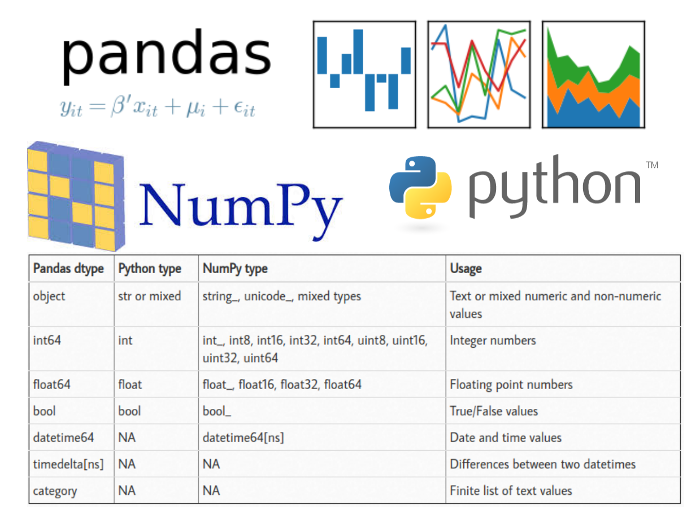
Python Data Types from Basic to Advanced
Python Data Types
1. Booleans
A boolean is such a data type that almost every programming language has, and so is Python. Boolean in Python can have two values – True or False. These values are constants and can be used to assign or compare boolean values. Follow a simple example given below.
condition = False
if condition == True:
print("You can continue with the prpgram.")
else:
print("The program will end here.")
While making boolean conditions in Python, we can skip the explicit comparison in our code. And we’ll still get the same behavior.
condition = False
if condition:
print("You can continue with the prpgram.")
else:
print("The program will end here.")
The above code will yield the same output as gave the previous one. It is because of the statement
if condition:
is equivalent to,
if condition == True:
Next, an expression in Python can also produce a boolean result.
For example – The expression in a condition block will yield a boolean value. Python creates boolean contexts to evaluate expressions.
Whatever be the expression is, Python will use the boolean context to determine its truth value. Since Python has many data types, so they will operate with their own rules to find the result in a boolean context.
>>> str = "Learn Python"
>>> len(str)
12
>>> len(str) == 12
True
>>> len(str) != 12
False
In some cases, the boolean constants “True” and “False” might also act as numbers.
>>> A, B = True + 0, False + 0
>>> print(A, B)
1 0
>>> type(A), type(B)
(<class 'int'>, <class 'int'>)
It is evident from the above example that True is 1 and the value of False is 0. And they will turn into numbers during arithmetic operations.
2. Numbers
Numbers are one of the most prominent Python data types. Unlike many languages which have only integers and floats, Python introduces complex as a new type of numbers.
Here are a few points for you to ponder.
The numbers in Python are classified using the following keywords.
int, float, and complex.
Python has a built-in function type() to determine the data type of a variable or the value.
Another built-in function isinstance() is there for testing the type of an object.
In Python, we can add a “j” or “J” after a number to make it imaginary or complex.
Example.
num = 2
print("The number (", num, ") is of type", type(num))
num = 3.0
print("The number (", num, ") is of type", type(num))
num = 3+5j
print("The number ", num, " is of type", type(num))
print("The number ", num, " is complex number?", isinstance(3+5j, complex))
#Output
The number ( 2 ) is of type <class 'int'>
The number ( 3.0 ) is of type <class 'float'>
The number (3+5j) is of type <class 'complex'>
The number (3+5j) is complex number? True
To form a complex number, we can even use the type as a constructor. See the example below.
>>> complex(1.2,5)
(1.2+5j)
Integers in Python don’t have any size limitation as long as the required memory is available.
>>> num = 1234567890123456789
>>> num.bit_length()
61
>>> num
1234567890123456789
>>> num = 1234567890123456789123456789012345678912345678901234567891234567890123456789
>>> num.bit_length()
250
>>> num
1234567890123456789123456789012345678912345678901234567891234567890123456789
A float type number can have precision up to 15 decimal places.
>>> import sys
>>> sys.float_info
sys.float_info(max=1.7976931348623157e+308, max_exp=1024, max_10_exp=308, min=2.2250738585072014e-308, min_exp=-1021, min_10_exp=-307, dig=15, mant_dig=53, epsilon=2.220446049250313e-16, radix=2, rounds=1)
>>> sys.float_info.dig
15
Note – The dig in above example is the maximum number of decimal digits in a float.
3. Strings
A sequence of one or more characters enclosed within either single quotes ‘ or double quotes ” is considered as String in Python. Any letter, a number or a symbol could be a part of the sting.
Python also supports multi-line strings which require a triple quotation mark at the start and one at the end.
>>> str = 'A string wrapped in single quotes'
>>> str
'A string wrapped in single quotes'
>>> str = "A string enclosed within double quotes"
>>> str
'A string enclosed within double quotes'
>>> str = """A multiline string
starts and ends with
a triple quotation mark."""
>>> str
'A multiline string\nstarts and ends with\na triple quotation mark.'
Also, the strings in Python are immutable. It means the memory will be allocated once and re-used thereafter.
>>> A = 'Python3'
>>> id(A)
56272968
>>> B = A
>>> id(B)
56272968
You can see the second string shares the same address as the first one does.
Python has two popular versions, namely 2.7 and 3.4. Most programmers around the globe use either of them. The strings in Python 2 are by default non-Unicode (ASCII) but also have support for Unicode.
On the other hand, Python 3 strings are all Unicode (UTF-8).
Strings in Python 2.
>>> print(type('Python String'))
<type 'str'>
>>> print(type(u'Python Unicode String'))
<type 'unicode'>
Strings in Python 3.
>>> print(type('Python String'))
<class 'str'>
>>> print(type(u'Python Unicode String'))
<class 'str'>
Python allows slicing strings using a special square-bracket syntax to extract a substring. See the example below.
>>> str = "Learn Python"
>>> first_5_chars = str[0:5]
>>> print(first_5_chars)
Learn
>>> substr_from_2_to_5 = str[1:5]
>>> print(substr_from_2_to_5)
earn
>>> substr_from_6_to_end = str[6:]
>>> print(substr_from_6_to_end)
Python
>>> last_2_chars = str[-2:]
>>> print(last_2_chars)
on
>>> first_2_chars = str[:2]
>>> print(first_2_chars)
Le
>>> two_chars_before_last = str[-3:-1]
>>> print(two_chars_before_last)
ho
4. Bytes
The byte is an immutable type in Python. It can store a sequence of bytes (each 8-bits) ranging from 0 to 255. Similar to an array, we can fetch the value of a single byte by using the index. But we can not modify the value.
Here are a few differences between a byte and the string.
Byte objects contain a sequence of bytes whereas the strings store sequence of characters.
The bytes are machine-readable objects whereas the strings are just in human-readable form.
Since the byte is machine-readable, so they can be directly stored to the disk. Whereas, the strings first need to encoded before getting on to the disk.
>>> # Make an empty bytes object (8-bit bytes)
>>> empty_object = bytes(16)
>>> print(type(empty_object))
<class 'bytes'>
>>> print(empty_object)
b'\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00\x00'
One scenario, where bytes matter is when carrying out I/O operations with buffering enabled. For example, we have a program which is continuously receiving the data over the network. It parses the date after waiting for the message headers and terminators to appear in the stream. It keeps appending the incoming bytes to a buffer.
With Python byte object, it is easy to program the above scenario using the below pseudo code.
buf = b''
while message_not_complete(buf):
buf += read_from_socket()
5. Lists
Python list is an array like construct which stores arbitrarily typed objects in an ordered sequence. It is very flexible and does not have a fixed size. Index in a list begins with zero in Python.
It is a heterogeneous collection of items of varied data types. For example, a list object can store the files in a folder, or the employee data in a company etc.
Python list syntax
Lists in Python can be declared by placing elements inside square brackets separated by commas.
>>> assorted_list = [True, False, 1, 1.1, 1+2j, 'Learn', b'Python']
>>> first_element = assorted_list[0]
>>> print(first_element)
True
>>> print(assorted_list)
[True, False, 1, 1.1, (1+2j), 'Learn', b'Python']
>>> for item in assorted_list:
print(type(item))
<class 'bool'>
<class 'bool'>
<class 'int'>
<class 'float'>
<class 'complex'>
<class 'str'>
<class 'bytes'>
List objects are mutable. Python allows modifying a list or its elements via assignments as well as through the built-in list methods.
>>> simpleton = ['Learn', 'Python', '2']
>>> id(simpleton)
56321160
>>> simpleton
['Learn', 'Python', '2']
>>> simpleton[2] = '3'
>>> id(simpleton)
56321160
>>> simpleton
['Learn', 'Python', '3']
Nesting inside a list
Interestingly, a list can contain another list. Such a list is called as the nested list.
>>> nested = [[1,1,1], [2,2,2], [3,3,3]]
>>> for items in nested:
for item in items:
print(item, end=' ')
1 1 1 2 2 2 3 3 3
Slicing a list
The list is also one of the Python data types which supports slicing like we learned previously with Strings. With the slicing operator [ ], we can extract an element or a bunch of them from a list.
>>> languages = ['C', 'C++', 'Python', 'Java', 'Go', 'Angular']
>>> print('languages[0:3] = ', languages[0:3])
languages[0:3] = ['C', 'C++', 'Python']
>>> print('languages[2:] = ', languages[2:])
languages[2:] = ['Python', 'Java', 'Go', 'Angular']
6. Tuples
A tuple is a heterogeneous collection of Python objects separated by commas. It means objects of different data types can co-exist in a tuple. The tuple and a list are somewhat similar as they share the following traits.
Both objects are an ordered sequence.
They enable indexing and repetition.
Nesting is allowed.
They can store values of different types.
Python tuple syntax
Define a tuple using enclosing parentheses () having its elements separated by commas inside.
Example – Define a tuple
# Defining a tuple without any element
pure_tuple = ()
print (pure_tuple)
# Output- ()
Example – Nested tuples
# Creating a tuple with nested tuples
first_tuple = (3, 5, 7, 9)
second_tuple = ('learn', 'python 3')
nested_tuple = (first_tuple, second_tuple)
print(nested_tuple)
# Output - ((3, 5, 7, 9), ('learn', 'python 3'))
Example – Repetition in tuples
# How does repetition work with tuples
sample_tuple = ('Python 3',)*3
print(sample_tuple)
# Output - ('Python 3', 'Python 3', 'Python 3')
Example – Slicing in tuples
# How does slicing work with tuples
sample_tuple = (0 ,1, 2, 3, 4)
tuple_without_first_item = sample_tuple[1:]
print(tuple_without_first_item)
tuple_reverse = sample_tuple[::-1]
print(tuple_reverse)
tuple_from_3_to_5 = sample_tuple[2:4]
print(tuple_from_3_to_5)
# Output -
(1, 2, 3, 4)
(4, 3, 2, 1, 0)
(2, 3)
Important note – While slicing in the above example, The “2” means to start at the third element in the tuple (the slicing index begins at 0). The “4” means to end at the fifth element in the tuple but to exclude it.
How does a tuple differ from the list?
Tuples do differ a bit from the list as they are immutable. Python does not allow to modify a tuple after it is created. We can not add or remove any element later. Instead, Python expects us to create a new one with the updated sequence of elements.
What if a tuple has mutable objects as elements?
Here, comes the surprise. Modifying a tuple is forbidden. But Python doesn’t enforce it on the elements. It means we can update them if they are mutable objects.
Why need a Tuple as one of the Python data types?
Here are a couple of thoughts in support of tuples.
Python uses tuples to return multiple values from a function.
Tuples are more lightweight than lists.
It works as a single container to stuff multiple things.
We can use them as a key in a dictionary.
7. Sets
Amongst all the Python data types, the set is one which supports mathematical operations like union, intersection, symmetric difference etc.
A set is an unordered collection of unique and immutable objects. Its definition starts with enclosing braces { } having its items separated by commas inside.
Since the set derives its implementation from the “Set” in mathematics, so it can’t have multiple occurrences of the same element.
Why need a set?
The set type has a significant advantage over a list. It implements a highly optimized method that checks whether the container hosts a specific element or not. The mechanism used here is based on a data structure known as a hash table.
Creating a set
To create a set, call the built-in set() function with a sequence or any iterable object.
>>> sample_set = set("Python data types")
>>> type(sample_set)
<class 'set'>
>>> sample_set
{'e', 'y', 't', 'o', ' ', 'd', 's', 'P', 'p', 'n', 'h', 'a'}
Another simpler way is to specify the elements enclosed in curly braces {}.
>>> another_set = {'red', 'green', 'black'}
>>> type(another_set)
<class 'set'>
>>> another_set
{'red', 'green', 'black'}
Frozen set
A frozen set is a processed form of the traditional set. It is immutable and only supports methods and operators that executes without altering the frozen set used in the context.
# An empty frozenset
>>> frozenset()
frozenset()
>>> cities = {"New York City", "Saint Petersburg", "London", "Munich", "Paris"}
>>> fset = frozenset(cities)
>>> type(fset)
<class 'frozenset'>
Now, see a full example to highlight the difference between a normal and the frozen set.
# Python program to demonstrate frozen set
# A standard set
sample_set = {"red", "green"}
# Add an element to the standard set
sample_set.add("black")
print("Standard Set")
print(sample_set)
# A frozen set
frozen_set = frozenset(["red", "green", "black"])
print("Frozen Set")
print(frozen_set)
# Output -
Standard Set
{'green', 'red', 'black'}
Frozen Set
frozenset({'green', 'red', 'black'})
8. Dictionaries
A dictionary in Python is an unordered collection of key-value pairs. It’s a built-in mapping type in Python where keys map to values. These key-value pairs provide an intuitive way to store data.
Why need a dictionary?
The dictionary solves the problem of efficiently storing a large data set. Python has made the dictionary object highly optimized for retrieving data.
Creating a dictionary
Python syntax for creating dictionaries use braces {} where each item appears as a pair of keys and values. The key and value can be of any Python data types.
>>> sample_dict = {'key':'value', 'jan':31, 'feb':28, 'mar':31}
>>> type(sample_dict)
<class 'dict'>
>>> sample_dict
{'mar': 31, 'key': 'value', 'jan': 31, 'feb': 28}
Accessing dictionaries elements with keys
Dictionaries act like a database. Here, we don’t use a number to get a particular index value as we do with a list. Instead, we replace it with a key and then use the key to fetch its value.
>>> sample_dict['jan']
31
>>> sample_dict['feb']
28
Dictionaries methods to access elements
Python exposes following built-in dictionary functions.
keys() – It isolates the keys from a dictionary.
values() – It isolates the values from a dictionary.
items() – It returns the items in a list style of (key, value) pairs.
>>> sample_dict.keys()
dict_keys(['mar', 'key', 'jan', 'feb'])
>>> sample_dict.values()
dict_values([31, 'value', 31, 28])
>>> sample_dict.items()
dict_items([('mar', 31), ('key', 'value'), ('jan', 31), ('feb', 28)])
Modifying a dictionary (Add/update/delete)
Since the dictionary object is mutable, so we can call add, update and delete operations on a dictionary object.
See the below example for more clarity on how to modify a dictionary.
>>> sample_dict['feb'] = 29
>>> sample_dict
{'mar': 31, 'key': 'value', 'jan': 31, 'feb': 29}
>>> sample_dict.update({'apr':30})
>>> sample_dict
{'apr': 30, 'mar': 31, 'key': 'value', 'jan': 31, 'feb': 29}
>>> del sample_dict['key']
>>> sample_dict
{'apr': 30, 'mar': 31, 'jan': 31, 'feb': 29}
Comments